Hello Developers
Welcome to the YNAB API!
(If you aren't a developer or you have no idea what an "API" is and you just want to sign in to your YNAB account, you can do that here.)
The YNAB API is REST based, uses the JSON data format and is secured with HTTPS. You can use it to build a personal application to interact with your own budget or build an application that any other YNABer can authorize and use. Be sure to check out what other YNABers have built in the Works with YNAB section and let us know when you build something yourself!
You can check our changelog to find out about updates and improvements to the API.
If you need support, please send an email to api@ynab.com.
Quick Start
If you're the type of person who just wants to get up and running as quickly as possible and then circle back to fill in the gaps, these steps are for you:
- Sign in to the YNAB web app and go to the "Account Settings" page and then to the "Developer Settings" page.
- Under the "Personal Access Tokens" section, click "New Token", enter your password and click "Generate" to get an access token.
- Open a terminal window and run this:
curl -H "Authorization: Bearer <ACCESS_TOKEN>" https://api.ynab.com/v1/budgets
You should get a response that looks something like this:
HTTP/1.1 200 OK Content-Type: application/json; charset=utf-8 {"data": { "budgets": [ { "id":"6ee704d9-ee24-4c36-b1a6-cb8ccf6a216c", "name":"My Budget", "last_modified_on":"2017-12-01T12:40:37.867Z", "first_month":"2017-11-01", "last_month":"2017-11-01" } ] } }
That's it! You just received a list of your budgets in JSON format through the YNAB API. Hooray!
If you want to start working with the API to build something more substantial, you might want to check out our YNAB API Starter Kit which is a simple, but functional web application that uses the API.
Authentication
Overview
All API resources require a valid access token for authentication. There are two ways to obtain access tokens: Personal Access Tokens and OAuth Applications.
Personal Access Tokens
Personal Access Tokens are access tokens created by an account owner and are intended to be used only by that same account owner. They should not be shared and are intended for individual usage scenarios. They are a convenient way to obtain an access token without having to use a full OAuth authentication flow. If you are an individual developer and want to simply access your own account through the API, Personal Access Tokens are the best choice.
Obtaining a Personal Access Token
To obtain a Personal Access Token, sign in to your account, go to "Account Settings", scroll down and navigate to "Developer Settings" section. From the Developer Settings page, click "New Token" under the Personal Access Tokens section, enter your password and you will be presented with a new Personal Access Token. You will not be able to retrieve the token later so you should store it in a safe place. This new token will not expire but can be revoked at any time from this same screen.
You should not share this access token with anyone or ask for anyone else's access token. It should be treated with as much care as your main account password.
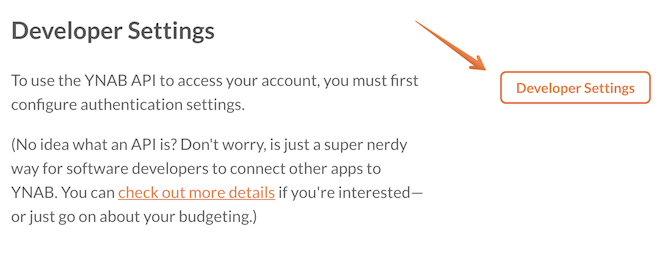
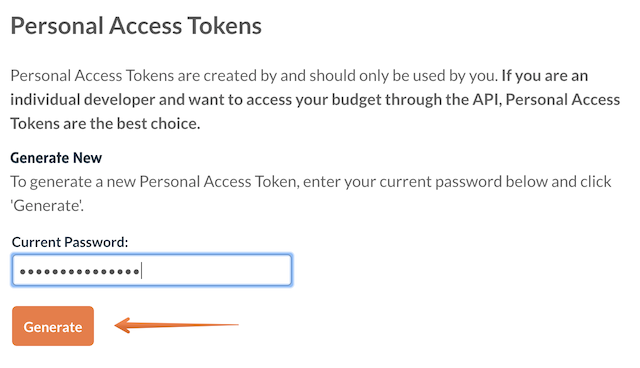
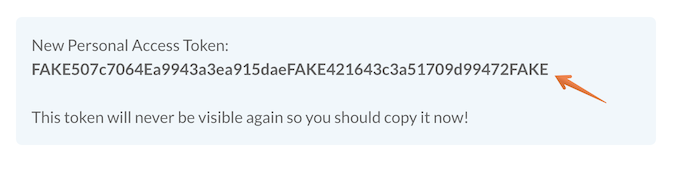
OAuth Applications
OAuth is a secure way for a third-party application to obtain delegated but limited permissions to a user account and is appropriate for use in applications that need to gain limited authorized permissions to accounts they do not own. If you are developing an application that uses the API and want other users to be able to use your application, OAuth is the only option for obtaining access tokens for other users.
All OAuth Application integrations must abide by the API Terms of Service and the OAuth Application Requirements. Failure to do so will result in disabling of the application.
Restricted Mode
When an OAuth application is created, it will be placed in Restricted Mode initially. This means the application will be limited to obtaining 25 access tokens for users other than the OAuth application owner. Once this limit is reached, a message will be placed on the Authorization screen and new authorizations will be prohibited.
To have Restricted Mode removed, you must send a request to api@ynab.com. We will review your OAuth application to ensure it abides by the API Terms of Service and the OAuth Application Requirements. Once we review the application and confirm adherence to our policies, we will remove Restricted Mode.
Creating an OAuth Application
To create an OAuth Application, sign in to your account, go to "Account Settings", scroll down and navigate to "Developer Settings" section. From the Developer Settings page, click "New Application" under the OAuth Applications section. Here, you specify the details of your application and save it.
After saving, you will see the details of the new application, including the Client ID and the Client Secret which are referenced in the instructions below.
After creating the application, you are then able to use one of the supported grant types to obtain a valid access token. The YNAB API supports two OAuth grant types: Implicit Grant and Authorization Code Grant.
Implicit Grant Flow
The Implicit Grant type, also informally known as the "client-side flow", should be used in scenarios where the application Secret cannot be kept private. The application Secret should never be visible or accessible by a client! If you are requesting an access token directly from a browser or other client that is not secure (i.e. mobile app) this is the flow you should use. This grant type does not support refresh tokens so once the access token expires 2 hours after it was granted, the user must be prompted again to authorize your application.
The YNAB API Starter Kit implements the Implicit Grant Flow and can be a good starting point for your own project or used as a reference for implementing OAuth.
Here is the flow to obtain an access token:
- Send user to the authorization URL:
https://app.ynab.com/oauth/authorize?client_id=[CLIENT_ID]&redirect_uri=[REDIRECT_URI]&response_type=token
, replacing [CLIENT_ID] and [REDIRECT_URI] with the values configured when creating the OAuth Application. The user will be given the option to approve your request for access: - Upon approval, the user's browser will be redirected to the [REDIRECT_URI] with a new access token sent as a fragment (hash) identifier named access_token. For example, if your Redirect URI is configured as https://myawesomeapp.com, upon the user
authorizing your application, they would be redirected to
https://myawesomeapp.com/#access_token=8bc63e42-1105-11e8-b642-0ed5f89f718b
. This access token can then be used to authenticate through the API.
Authorization Code Grant Flow
The Authorization Code Grant type, also informally known as the "server-side flow", is intended for server-side applications, where the application Secret can be protected. If you are requesting an access token from a server application that is private and under your control, this grant type can be used. This grant type supports refresh tokens so once the access token expires 2 hours after it was granted, the application can request a new access token without having to prompt the user to authorize again.
Here is the flow to obtain an access token:
- Send user to the authorization URL:
https://app.ynab.com/oauth/authorize?client_id=[CLIENT_ID]&redirect_uri=[REDIRECT_URI]&response_type=code
, replacing [CLIENT_ID] and [REDIRECT_URI] with the values configured when creating the OAuth Application. The user will be given the option to approve your request for access: - Upon approval, the user's browser will be redirected to the [REDIRECT_URI] with a new authorization code sent as a query parameter named code. For example, if your Redirect URI is configured as https://myawesomeapp.com, upon the user
authorizing your application, they would be redirected to
https://myawesomeapp.com/?code=8bc63e42-1105-11e8-b642-0ed5f89f718b
. -
Now that your application has an authorization code, you need to request an access token by sending a POST request to
https://app.ynab.com/oauth/token?client_id=[CLIENT_ID]&client_secret=[CLIENT_SECRET]&redirect_uri=[REDIRECT_URI]&grant_type=authorization_code&code=[AUTHORIZATION_CODE]
. Here are the values that should be passed as form data fields:- client_id - The same [CLIENT_ID] sent with the original authorization code in Step 1 above and provided when setting up the OAuth Application.
- client_secret - The client secret provided when setting up the OAuth Application.
- redirect_uri - The same [REDIRECT_URI] sent with the original authorization code request in Step 1 above and specified when setting up the OAuth Application.
- grant_type - The value
authorization_code
should be provided for this field, which will indicate that you are supplying an authorization code and requesting an access token. - code - The authorization code received as code query param in Step 2 above.
The access_token, which can be used to authenticate through the API, will be included in the token response which will look like this:
{ "access_token": "0cd3d1c4-1107-11e8-b642-0ed5f89f718b", "token_type": "bearer", "expires_in": 7200, "refresh_token": "13ae9632-1107-11e8-b642-0ed5f89f718b" }
- The access token has an expiration, indicated by the "expires_in" value. To obtain a new access token without requiring the user to manually authorize again, you should store the "refresh_token" and then send a POST request to
https://app.ynab.com/oauth/token?client_id=[CLIENT_ID]&client_secret=[CLIENT_SECRET]&grant_type=refresh_token&refresh_token=[REFRESH_TOKEN]
. If successful, the response will contain a new access token and a new refresh token in the original token response format. A refresh token can only be used once to obtain a new access token, and will be revoked the first time you use the new access token.
Authorization Parameters
read-only Scope
When an OAuth application is requesting authorization, a scope
parameter with a value of read-only
can be passed to request read-only access to a budget.
For example: https://app.ynab.com/oauth/authorize?client_id=[CLIENT_ID]&redirect_uri=[REDIRECT_URI]&response_type=token&scope=read-only
. If an access token issued with the read-only
scope is used when requesting an endpoint that modifies the budget (POST, PATCH, etc.) a 403 Forbidden
response will be issued.
If you do not need write access to a budget, please use the read-only scope.
state parameter
An optional, but recommended, state
parameter can also be supplied to prevent Cross Site Request Forgery (XRSF) attacks. If specified, the same value will be returned to the [REDIRECT_URI] as a state
parameter. For example:
if you included parameter state=4cac8f43
in the authorization URI, when the user is redirected to [REDIRECT_URI], the URL would contain that same value in a state
parameter. The value of state
should be unique for each request.
Default Budget Selection
An OAuth application can optionally have 'default budget selection' enabled.

When default budget selection is enabled, a user will be asked to select a default budget when authorizing your application:

You can then pass in the value 'default' in lieu of a budget_id
in endpoint calls. For example, to get a list of accounts on the default budget you could use this endpoint: https://api.ynab.com/v1/budgets/default/accounts
.
Access Token Usage
Once you have obtained an access token, you must use HTTP Bearer Authentication, as defined in RFC6750, to authenticate when sending requests to the API. We support Authorization Request Header and URI Query Parameter as means to pass an access token.
Authorization Request Header
The recommended method for sending an access token is by using an Authorization Request Header where the access token is sent in the HTTP request header.
curl -H "Authorization: Bearer <ACCESS_TOKEN>" https://api.ynab.com/v1/budgets
URI Query Parameter
An access token can also be passed as a URI query parameter named "access_token":
curl https://api.ynab.com/v1/budgets?access_token=<ACCESS_TOKEN>
Usage
Overview
Our API uses a REST based design, leverages the JSON data format, and relies upon HTTPS for transport. We respond with meaningful HTTP response codes and if an error occurs, we include error details in the response body. We support Cross-Origin Resource Sharing (CORS) which allows you to use the API directly from a web application.
Mostly read-only
The current version of the API ("v1") is mostly read-only, supporting GET
requests. However, we do support some `POST`, `PATCH`, and `DELETE` requests on several resources. Take a look at the API Endpoints for details.
Security
TLS (a.k.a. SSL or HTTPS) is enforced on all requests to ensure communication from your client to our endpoint is encrypted, end-to-end. You must obtain an access token and provide it with each request. An access token is tied directly to a YNAB account but can be independently revoked.
Best Practices
Caching
Please cache data received from the API when possible to avoid unnecessary traffic.
Delta Requests
Some endpoints support Delta Requests, where you can request to receive only what has changed since the last response. It is highly recommended to use this feature as it reduces load on our servers as well as makes processing responses more efficient.
Fault Tolerance
Errors and exceptions will sometimes happen. You might experience a connection problem between your app and the YNAB API or a complete outage. You should always anticipate that errors or exceptions may occur and build in accommodations for them in your application.
Specific Requests
You should use the most specific request possible to avoid large responses which are taxing on the API server and
slower for your app to consume and process. For example, if you want to retrieve the balance for a particular
category, you should request that single category from /budgets/{budget_id}/categories/{category_id}
rather than requesting all categories.
Endpoints
The base URL is:
https://api.ynab.com/v1
. To see a list of all available endpoints, please refer to our API Endpoints page. The documentation also lets you "try it out" on each endpoint directly within the page.
Note: The YNAB API was previously available at https://api.youneedabudget.com/v1
. In 2023 it moved to https://api.ynab.com/v1
. While existing applications using https://api.youneedabudget.com/v1
will continue to function, all API consumers should be updated to use https://api.ynab.com/v1
.
Response Format
All responses from the API will come with a response wrapper object to make them predictable and easier to parse.
Successful Responses
Successful responses will return wrapper object with a data
property that will contain the resource data.
The name of the object inside of the data property will correspond to the requested resource.
For example, if you request /budgets
, the response will look like:
{ "data": { "budgets ": [ {"id": "cee64af3-a3df-425e-a18a-980e7ec10dc2", ...}, {"id": "55697d98-b942-4f29-97d8-f870edd001d6", ...} ] }
If you request a single account from /accounts/{account_id}
:
{ "data": { "account": {"id": "16da87a0-66c7-442f-8216-a3daf9cb82a8", ...} }
Empty data
Response data properties that have no data will be specified as null
rather than being omitted. For example, a transaction that does not have a payee would have a body that looks like this:
{ "data": { "transaction": { "id": "16da87a0-66c7-442f-8216-a3daf9cb82a8", "date": "2017-12-01", "payee_id": null, // This transaction does not have a payee ... } }
Error Responses
For error responses, the HTTP Status Code will be specified as something other than 20X
and the body of the response will contain an error object.
The format of an error response is:
{ "error": { "id": "123" "name": "error_name" "detail": "Error detail" } }
The Errors section lists the possible errors.
Errors
Errors from the API are indicated by the HTTP response status code and also included in the body of the response, according to the response format. The following errors are possible:
HTTP Status | Error ID | Name | Description |
---|---|---|---|
400 | 400 | bad_request | The request could not be understood by the API due to malformed syntax or validation errors. |
401 | 401 | not_authorized |
This error will be returned in any of the following cases:
|
403 | 403.1 | subscription_lapsed | The subscription for this account has lapsed |
403.2 | trial_expired | The trial for this account has expired | |
403.3 | unauthorized_scope | The supplied access token has a scope which does not allow access. | |
403.4 | data_limit_reached | The request will exceed one or more data limits in place to prevent abuse. | |
404 | 404.1 | not_found | The specified URI does not exist |
404.2 | resource_not_found | This error will be returned when requesting a resource that is not found. For example, if you requested /budgets/123 and a budget with the id '123' does not exist, this error would be returned. | |
409 | 409 | conflict | If resource cannot be saved during a PUT or POST request because it conflicts with an existing resource, this error will be returned. |
429 | 429 | too_many_requests | This error is returned if you make too many requests to the API in a short amount of time. Please see the Rate Limiting section. Wait awhile and try again. |
500 | 500 | internal_server_error | This error will be returned if the API experiences an unexpected error. |
503 | 503 | service_unavailable | This error will be returned if we have temporarily disabled access to the API. This can happen when we are experiencing heavy load or need to perform maintenance. |
Data Formats
Numbers
Currency amounts returned from the API—such as account balance, category balance, and transaction amounts— use a format we call "milliunits". Most currencies don't have three decimal places, but you can think of it as the number of thousandths of a unit in the currency: 1,000 milliunits equals "one" unit of a currency (one Dollar, one Euro, one Pound, etc.). Here are some concrete examples:
Currency | Milliunits | Amount |
---|---|---|
USD ($) | 123930 | $123.93 |
USD ($) | -220 | -$0.22 |
Euro (€) | 4924340 | €4.924,34 |
Euro (€) | -2990 | -€2,99 |
Jordanian dinar | -395032 | -395.032 |
Dates
All dates returned in response calls use ISO 8601 (RFC 3339 "full-date") format. For example,
December 30, 2015 is formatted as 2015-12-30
.
Timezone
All dates use UTC as the timezone.
Delta Requests
The following API resources support "delta requests", where you ask for only those entities that have changed since your last request:
GET /budgets/{budget_id}
GET /budgets/{budget_id}/accounts
GET /budgets/{budget_id}/categories
GET /budgets/{budget_id}/months
GET /budgets/{budget_id}/payees
GET /budgets/{budget_id}/transactions
GET /budgets/{budget_id}/scheduled_transactions
We recommend using delta requests as they allow API consumers to parse less data and make updates more efficient, and decreases server load on our end.
Resources supporting delta requests return a server_knowledge number in the response. This number can then be passed in as the last_knowledge_of_server query parameter. Then, only the data that has changed since the last request will be included in the response.
For example, if you request a budget's contents from
/budgets/{budget_id}
, it will include the
server_knowledge like so:
{ "data": { "server_knowledge": 100, "budget": { "id": "16da87a0-66c7-442f-8216-a3daf9cb82a8", ... } } }
On a subsequent request, you can pass that same
server_knowledge in as a query parameter named
last_knowledge_of_server
(/budgets/{budget_id}?last_knowledge_of_server=100
)
and get back only the entities that have changed since your
last request. For example, if a single account had its name changed
since your last request, the response would look something like:
{ "data":{ "server_knowledge":101, "budget":{ ... "accounts":[ { "id":"ea0c0ace-1a8c-4692-9e1d-0a21fe67f10a", "name":"Renamed Checking Account", "type":"Checking", "on_budget":true, "closed":false, "note":null, "balance":20000 } ], ... } } }
Rate Limiting
An access token may be used for up to 200 requests per hour.
The limit is reset every clock hour. So, if an access token is used at 12:30 PM and for 199 more requests up to 12:45 PM and then hits the limit, any additional requests will be forbidden until 1:00 PM. At 1:00 PM you would have the full 200 requests allowed again, until 2:00 PM.
You can check how many requests you have remaining by referencing the X-Rate-Limit
response header.
The value of this header is in the format: X/200
, X being the number of requests already made and 200 being the limit.
For example, if your application has already made 35 requests, the next response will look like this:
HTTP/1.1 200 OK X-Rate-Limit: 36/200 ...
If you exceed the rate limit, an error response returns a 429 error:
HTTP/1.1 429 Too Many Requests Content-Type: application/json; charset=utf-8 "error": { "id": "429" "name": "too_many_requests" "detail": "Too many requests" }
Support
If you need support, please send an email to api@ynab.com.
Libraries
JavaScript
The JavaScript library is available via npm and the source and documentation is located on GitHub. This library can be used server-side (Node.js) or client-side (web browser) since we support Cross-Origin Resource Sharing (CORS).
If you are using the JavaScript library, you might want to also take a look at the YNAB API Starter Kit which is a simple, but functional web application that uses the JavaScript library.
Installation
npm install ynab
Usage
const ynab = require("ynab"); const accessToken = "123-yourAccessTokenHere-456"; const ynabAPI = new ynab.API(accessToken); (async function() { const budgetsResponse = await ynabAPI.budgets.getBudgets(); const budgets = budgetsResponse.data.budgets; for(let budget of budgets) { console.log(`Budget Name: ${budget.name}`); } })();
Ruby
The Ruby library is available via RubyGems and the source and documentation is located on GitHub.
Installation
gem install ynab
If using Bundler, add gem 'ynab'
to your Gemfile and then run bundle
.
Usage
require 'ynab' access_token = '123-yourAccessTokenHere-456' ynab_api = YNAB::API.new(access_token) budget_response = ynab_api.budgets.get_budgets budgets = budget_response.data.budgets budgets.each do |budget| puts "Budget Name: #{budget.name}" end
Community
The following libraries have been created and are maintained by YNABers. We do not provide support for these clients but appreciate the effort!
- .NET
- YNAB.Rest by Joshua Marble
- YNAB API .NET Core Library by Jake Moening
- Go
- Java - Java SDK for YNAB API by David Dietz
- Julia - ynab-tools by Joseph Peralta
- Kotlin - kYNAB by Mark Nenadov
- Perl - WWW::YNAB by Jesse Luehrs
- PHP - YNAB-SDK-PHP by Jorijn Schrijvershof
- PowerShell - Posh-YNAB by Connor Griffin
- Python - ynab-python by Dean Mcgregor
- R - rnab by Eric Kort
- Rust - ynab-rs by Taryn Phrohdoh
- Swift - swiftynab by Andre Bocchini
Works with YNAB
Official
The following applications are official YNAB integrations that we developed and support.
- Alexa for YNAB - Gain More Control of Your Money (By Yelling at It)
- API Starter Kit - Quickly get started developing an application with the YNAB API
- Zapier for YNAB - Zapier allows you to instantly connect YNAB with 1,500+ apps to automate your work and find productivity super powers.
Community
The following community applications have been developed by fellow YNABers. We do not provide support for these applications.
- Additional Budget Graphs - Some additional graphing and other abilities for YNAB
- Advanced Tools For YNAB - Advanced Tools for YNAB gives you deep insights into your spending habits and gives you the tools needed to supercharge your YNAB budget.
- AECOM Global Well-Being - The AECOM online Global Well-Being platform
- Allowance for YNAB - An iOS app that allows a focused view of budget categories you pick. This can be useful for family members that only want to know how much they currently have available in a specific category or set of categories.
- Apple Card for YNAB - Manual Apple Card imports gotcha down? This service automates the process making it as simple as possible to get those Apple Card transactions synced over to YNAB. Step 1: Email Apple Card monthly statements as CSV attachments to parse@applecardforynab.com. Step 2: Relax.
- Apple Card Transaction Screenshot For Ynab - This application allows users to easily import their Apple Card transactions into their YNAB budget. With just a few clicks, users can upload a screenshot of their Apple Card Latest Transactions section, and our app will use OCR technology to extract the transaction details and upload them directly to their YNAB account. Our app provides a fast and hassle-free way for YNAB users to keep their budget up-to-date, without the need for manual data entry or waiting until the end of the month
- Automanual for YNAB - Automanual helps automate common manual YNAB tasks such as importing Apple Card transactions.
- Bank Sync for YNAB - Sync your Australian bank accounts to YNAB!
- bar for YNAB - A simple macOS menu bar for quick access to key YNAB information like amount to be budgeted, age of money, income and what's budgeted.
- Beyond Rule 4 - Your Age of Money is ever rising. You're feeling in control of your money. Congratulations! What would you like to accomplish next?
- Budget Buddy - Companion app for YNAB for your partner or significant other to track the budget categories that they care about. Users can see categories, category balances, and category transactions for the current month.
- Budget Feeder - Sync your Australian bank transactions to YNAB! 148 Australian Banks and credit cards supported including Amex, ANZ, Westpac, NAB and CBA. No need to enter transactions manually, with automatic bank feeds your transactions sync seamlessly to your YNAB budget accounts every day. Let Budget Feeder do the hard work for you.
- Budget Reminders - Get reminders of you current budget category balances by time or location.
- Budget Reports - See reports based on your YNAB account data
- budgetsyncer.com - Connects European PSD2 compatible banks to ynab
- Causal - Causal replaces your spreadsheets and slide decks with a better way to perform calculations, visualize data, and communicate with numbers. Sign up for free.
- Cents for YNAB - iOS and Android app to seamlessly view your YNAB budget with charts to visualize your spending habits
- Coda Sync for YNAB - A Coda pack to sync your transactions into a doc
- Cost Sharing for YNAB - Conveniently manage a shared credit card or bank account in YNAB
- DAKboard - DAKboard is a customizable display for photos, calendar, news, weather and so much more!
- Dash for YNAB - Dashboard and reporting tools for YNAB.
- Exist Integrations - Exist Integrations allows the money spent in your YNAB budget be sent into your Exist Dashboard.
- Foreign Currency Accounts for YNAB - Manage multiple currency accounts in a single budget.
- GPT for YNAB (Unofficial) - AI assistant for You Need A Budget (YNAB), powered by ChatGPT. Not affiliated with or endorsed by YNAB. As with any AI, this assistant can hallucinate or be flat out wrong. DO NOT make financial decisions based on this assistant.
- Heatmap for YNAB - A report that displays your YNAB transactions in a heatmap style to get a bird's eye view of your budget.
- Insights for YNAB - A connector to get data from YNAB into Google Data Studio.
- Integrately - Integrately is a 1-click integration app that helps you in integrating your favourite apps in just 1 click.
- JSON Exporter for YNAB - This allows an easy download of your full budget in JSON form, directly from YNAB's API.
- Know Your Spending - Know Your Spending? Quiz Yourself to Find Out.
- Lumy - Lumy is a mobile application offering beautiful and configurable charts that give you insight into your spending habits.
- Multi-currency for YNAB - Manage YNAB accounts with multiple currencies in a single budget
- Peek for YNAB - A browser extension to check on your category and account balances in YNAB
- Pipedream - Integration platform for developers
- ProjectionLab Sync - Syncs YNAB account balances with ProjectionLab. Allows you to grow beyond Rule 4 with detailed projections and tax models.
- Reconcile for YNAB - Simplified flow to reconcile YNAB accounts, even on mobile.
- Repeating Export for YNAB - Download your scheduled repeating transactions as a table to CSV or Excel.
- See Your Budget - Visualize your YNAB budget in various useful ways.
- Sprout for YNAB - Quickly add a new transaction from whatever webpage you're on.
- Stats for YNAB - Discover money insights right in your device.
- Sync for YNAB - Connect your bank to YNAB
- Synci.io - Automatically import bank transactions to YNAB. Synci is connected to 2447 banks across 31 European countries.
- Target Visualizer for YNAB - Visualize your monthly targets to work out how much money you need to earn and where you've allocated your targets.
- Telegram bot for YNAB - A Telegram Messenger bot that allows you to quickly add transactions to your account and to import CSV files from banks that are not directly supported by YNAB.
- Transaction Resurrecter - Application for resurrecting deleted YNAB transactions.
- Undebt.it - Undebt.it is a free, mobile-friendly debt snowball calculator
- Widgets for YNAB - Display helpful YNAB information on your iOS Home Screen.
- Ytistic - A web app that aim to provide more reporting and statistics on your budgets and cashflows!
Legal
API Terms of Service
We provide the YNAB API so that YNAB-loving developers can make really cool projects and applications. We have some expectations and guidelines about how you’ll do that. Officially, these guidelines are our API Terms of Service because, well, that’s what they’re called. They work hand-in-hand with our general YNAB Terms of Service, and both apply to your use of the API. We appreciate you reading them carefully and, naturally, following them.
To keep the text here readable, we refer to the following as the “Terms”:
- the YNAB Terms of Service;
- the API Terms of Service below;
- terms within any API documentation;
- and any other applicable policies.
In order to protect the website, our apps, and our customers and their data, you agree to comply with them and that they govern your relationship with us.
With that said, here are the YNAB API Terms of Service:
- Authorized Use. To use the YNAB API and accept the Terms you must be of a legal age to form a binding contract with YNAB. The YNAB API may only be used when permission is explicitly given by a YNAB account owner through the Authentication processes described in the documentation above.
- Security and Permitted Access. Access tokens must be handled securely and never be exposed to a third-party. The Terms and API documentation outline the only permissible ways in which you can interact with the YNAB API. You are NEVER ALLOWED to directly request, handle or store credentials associated with users’ financial accounts. Securely storing an access token obtained directly from a financial institution using OAuth is allowed.
- API Limitations. YNAB sets and enforces limits on your use of the APIs at our discretion. Those limits may change and are at our sole discretion. Any attempt to circumvent those limitations is a violation of these terms.
- Illegal and Restricted Use. We developed this API so you can do good, kind, helpful things with it and to make YNAB better. So: The YNAB API may not be used for illegal purposes. Which seems obvious, but it’s important to say it. Beyond legality, we also restrict the use of the YNAB API in certain ways. You agree not to use, or allow any third party to use, the YNAB API to engage in or promote any activity that is objectionable, violates the rights of others, is likely to cause notoriety, harm or damage to the reputation of YNAB or could subject YNAB to liability to third parties. This might include: (i) unauthorized access, monitoring, interference with, or use of the YNAB API or third party accounts, data, computers, systems or networks; (ii) interference with others’ use of the YNAB API or any system or network; (iii) unauthorized collection or use of personal or confidential information; (iv) any other activity that places YNAB in the position of having potential or actual liability for activity in any jurisdiction.
- Attribution & Intellectual Property. You and your integration or app may not identify or refer to YNAB in any manner that creates a false suggestion (either directly or indirectly!) that an application is sponsored, endorsed, or supported by YNAB. This includes an application name, description, graphics and artwork, and/or web address (DNS name). To identify that your app integrates with YNAB, you may use this linked image and refer to “for YNAB” in the name of your application. Any other uses of our content are subject to the Intellectual Property Rights and Trademarks sections of the YNAB Terms of Service. Don’t use ‘em.
- Functionality and Non-exclusivity. You may not use the YNAB API to copy or duplicate products or services offered by YNAB. Also, you acknowledge that YNAB may, now or in the future, offer products, services, or features that are similar to your application.
- Compliance and Monitoring. YNAB may, but has no obligation to, monitor use of the YNAB API to verify your compliance with the Terms or any other applicable law or legal requirement.
- Accept Updates. The YNAB API may periodically be updated with tools, utilities, improvements, or general updates. You agree to receive these updates.
- Termination. YNAB may terminate or suspend any and all access to the API immediately at any time, without prior notice or liability at our sole discretion.
- Changes. We reserve the right to modify the Terms at any time by posting them on this page and/or notifying you by any means of contact you’ve provided. It is important that you review the Terms whenever we modify them because your continued use of the YNAB API indicates your agreement to the modifications.
OAuth Application Requirements
In addition to the above terms, OAuth Applications must adhere to these requirements:
- A Privacy Policy must be in place for the application that is displayed to users and that includes the following:
- An explanation of how the data obtained through the YNAB API will he handled, stored, and secured.
- A guarantee that the data obtained through the YNAB API will not unknowingly be passed to any third-party.
- The application must not directly request, handle or store any financial account credentials other than an access token obtained directly from a financial institution using OAuth.
-
In line with the Attribution & Intellectual Property section of the API Terms of Service above:
- The application and the web address (DNS name) must not include "YNAB" or "You Need A Budget" unless preceded by the word "for".
Acceptable: "Budget Tools", "Transaction Syncer", "Currency Tools for YNAB".
Unacceptable: "YNAB Tools", "YNAB Transaction Syncer", "Advanced YNAB". - Any graphics or artwork may not be modifications to our official branding and must be distinguishable from YNAB itself.
- The application and the web address (DNS name) must not include "YNAB" or "You Need A Budget" unless preceded by the word "for".
Changelog
v1.68.1
2024-04-24
Remove `server_knowledge` field from single category resource responses (`GET budgets/{budget_id}/months/{month}/categories/{category_id}` and `GET budgets/{budget_id}/categories/{category_id}`). These endpoints do not support delta requests and the `server_knowledge` field has been mistakenly included in their responses.
v1.68.0
2024-02-26
v1.1.0 - v1.67.0
2024-02-20
Various new features and enhancements.